AWS AppSync Overview: GraphQL, Resolvers, and Advanced Techniques
Dive deep into AWS AppSync, starting from the fundamentals of GraphQL such as queries, mutations, and subscriptions, to more advanced topics including AppSync resolvers, authorization, API logging, and caching techniques.
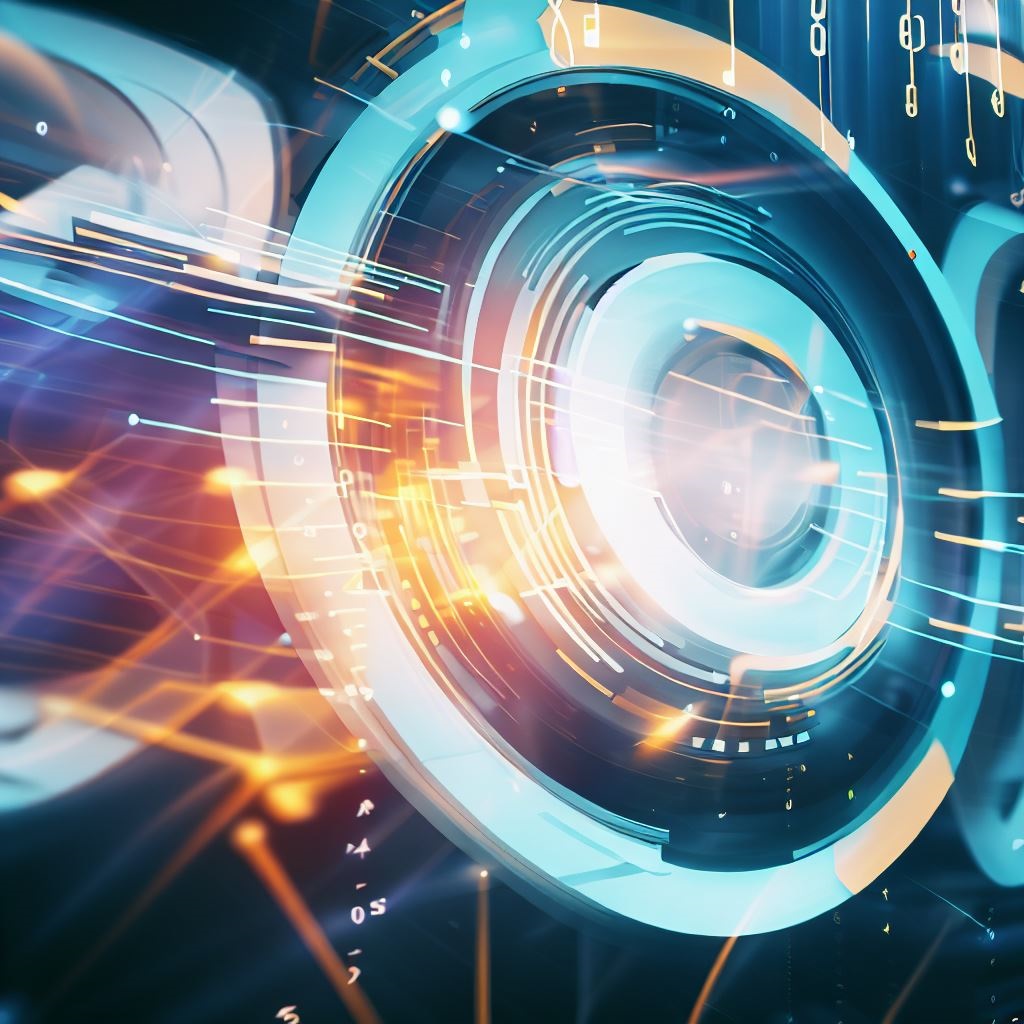
Table of Contents
Introduction:
AWS AppSync is a fully managed service offered by Amazon Web Services (AWS) that simplifies the development of serverless GraphQL APIs (Application Programming Interfaces). It enables developers to build scalable, real-time, and data-driven applications by providing a managed GraphQL service.
AWS AppSync is commonly used for building web and mobile applications that require real-time updates, complex data fetching, and offline capabilities. It abstracts much of the underlying infrastructure complexity, making it easier for developers to create powerful and responsive applications.
GraphQL:
Query
A GraphQL query is a request for data from a GraphQL API. It’s one of the fundamental operations in GraphQL and is used by clients to retrieve specific pieces of data from a server. GraphQL queries are expressive, client-defined, and allow clients to request only the data they need, which makes them efficient and flexible for various use cases
Mutation
GraphQL mutations are a type of GraphQL operation used for modifying data on the server. While GraphQL queries are used to request data, mutations are used to perform actions that create, update, or delete data. Mutations enable clients to make changes to the underlying data through a well-defined and structured process.
GraphQL mutations provide a structured and efficient way to perform data-changing operations on a GraphQL API. They follow the same principles of flexibility and client-driven requests as queries, making them a powerful tool for interacting with GraphQL APIs.
Subscription
GraphQL subscriptions are a feature of GraphQL that enable real-time data updates and event-driven communication between clients and servers. While GraphQL queries retrieve data and mutations modify data, subscriptions allow clients to receive data in response to specific events or changes on the server. This makes GraphQL a powerful tool for building interactive and dynamic applications, such as chat apps, live dashboards, and real-time notifications.
GraphQL subscriptions provide a standardized and efficient way to implement real-time features in applications. They enable developers to build interactive and engaging user experiences by keeping clients synchronized with the latest data changes on the server in a highly flexible and declarative manner.
Schema:
schema {
query: Query
mutation: Mutation
}
type Post {
id: ID!
title: String!
content: String!
author: User!
createdAt: String!
}
type Query {
# Retrieve a list of all posts
getAllPosts: [Post!]!
# Retrieve a specific post by its ID
getPostById(postId: ID!): Post
}
type Mutation {
# Create a new post
createPost(title: String!, content: String!, authorId: ID!): Post!
# Update an existing post
updatePost(postId: ID!, title: String, content: String): Post
# Delete a blog post by its ID
deletePost(postId: ID!): Boolean
}
AppSync Resolvers
Resolver:
An AWS AppSync resolver is a critical component of the AWS AppSync service that defines how data is fetched or manipulated from a data source in response to GraphQL requests. In simpler terms, it acts as a bridge between your GraphQL API and your backend data services.
A resolver’s primary function is to map GraphQL operations (queries, mutations, subscriptions) to specific actions on your data sources, which can include databases like AWS DynamoDB, AWS Lambda functions, HTTP endpoints, and more. Here’s a more detailed breakdown:
Resolvers are like bridges between your GraphQL API and data sources. They determine how GraphQL requests are translated into actions on your data sources.
- AWS AppSync uses mapping templates, written in a language called Apache Velocity Template Language (VTL), within resolvers to specify how data should be fetched or manipulated.
- These templates guide the process of fetching or updating data from the chosen data source when GraphQL queries or mutations are made.
- We have two types of AppSync resolvers (VTL -Velocity Template Language) and AppSync JS(Latest).
Automatic Provisioning:
- AWS AppSync can automatically set up DynamoDB tables based on your GraphQL schema, simplifying the process of creating data sources.
- You can also import existing DynamoDB tables into your API, which will automatically generate a GraphQL schema and connect resolvers to them.
- In summary, AWS AppSync uses data sources to fetch data and resolvers to determine how to interact with those data sources based on your GraphQL requests. It can automatically create data sources and resolvers for you, or you can configure them manually, depending on your needs. Mapping templates written in VTL are used to specify the exact behavior of resolvers.
Data Sources:
- Data sources are where AWS AppSync fetches information from. AWS AppSync supports different types of data sources, including AWS Lambda functions, Amazon DynamoDB, relational databases like Amazon Aurora Serverless, Amazon OpenSearch Service, and HTTP endpoints.
- You can use existing AWS resources as data sources or create new ones specifically for your GraphQL API.
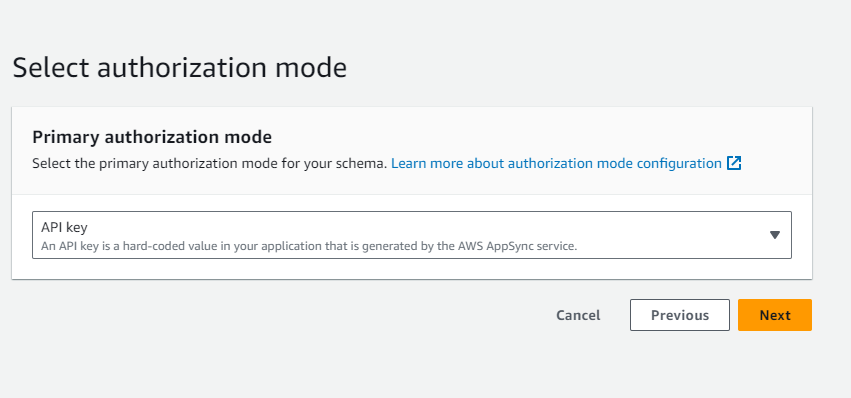
Resolver Types
- DynamoDB Resolvers:
- These resolvers connect your GraphQL API to AWS DynamoDB tables.
- You can define how queries and mutations in your API interact with DynamoDB, like fetching, creating, updating, or deleting records.
- Lambda Resolvers:
- Lambda resolvers connect your API to AWS Lambda functions.
- You can use Lambda functions to handle custom logic or integrate with other AWS services in response to GraphQL requests.
- Amazon OpenSearch Service Resolvers:
- These resolvers enable you to search and retrieve data from Amazon OpenSearch Service (formerly known as Elasticsearch).
- You define how your GraphQL queries interact with OpenSearch for full-text search and analytics.
- Local Resolvers:
- Local resolvers let you manage and query data stored locally on your client devices.
- Useful for building offline-capable applications where data is stored locally and then synchronized when online.
- Combining GraphQL Resolvers:
- You can combine multiple resolvers to create complex data fetching logic.
- This is helpful when you need to fetch data from multiple data sources or perform multiple operations in response to a single GraphQL request.
- DynamoDB Batch Resolvers:
- These resolvers allow you to perform batch operations on DynamoDB tables, like fetching multiple records in a single request for efficiency.
- DynamoDB Transaction Resolvers:
- Transaction resolvers enable you to perform multiple DynamoDB operations as a single, atomic transaction, ensuring data consistency.
- HTTP Resolvers:
- HTTP resolvers connect your GraphQL API to external HTTP endpoints, like REST APIs.
- Useful for integrating with external services that use HTTP for communication.
- Aurora Serverless:
- These resolvers connect your GraphQL API to Amazon Aurora Serverless relational databases.
- You can define how queries and mutations interact with Aurora Serverless for structured data storage.
- Pipeline Resolvers:
- Pipeline resolvers allow you to execute a sequence of resolvers in a specific order.
- You can use them to build advanced data processing pipelines for your GraphQL API.
- Delta Sync:
- Delta Sync is a feature that helps you efficiently synchronize data changes between the server and clients.
- It allows clients to fetch only the changes since their last synchronization, reducing data transfer.
- Each type of resolver in AWS AppSync serves a specific purpose and allows you to connect your GraphQL API to different data sources or implement various data processing and synchronization scenarios, depending on your application’s needs.
AppSync Subscriptions
- AWS AppSync lets you use subscriptions for real-time updates in your applications, like live updates or push notifications.
- When clients use GraphQL subscription operations, AWS AppSync takes care of setting up and maintaining a secure WebSocket connection.
- This connection allows data to flow in real time from your data source to subscribers.
- AWS AppSync handles the technical details, like managing connections and scaling, so you can focus on building your application.
- Subscriptions in AWS AppSync are like real-time updates triggered by mutations (data changes).
- You can make any data source in real-time by adding a special instruction in your GraphQL schema on a mutation.
- AWS Amplify client libraries make it easy to manage these real-time connections automatically.
- These connections use WebSockets, which are a type of network protocol, to communicate between clients and the service.
- Subscriptions are triggered by mutations, and they send the changes to subscribers.
To get started with subscriptions, you must add a subscription entry point to your schema as follows:
Schema:
schema {
query: Query
mutation: Mutation
subscription: Subscription
}
type Subscription {
OnCreatePost: Post
@aws_subscribe(mutations: [“createPost”])
}
Authorization Types
There are five ways you can authorize applications to interact with your AWS AppSync GraphQL API. You specify which authorization type you use by specifying one of the following authorization type values in your AWS AppSync API or CLI call:
API_KEY:For using API keys.
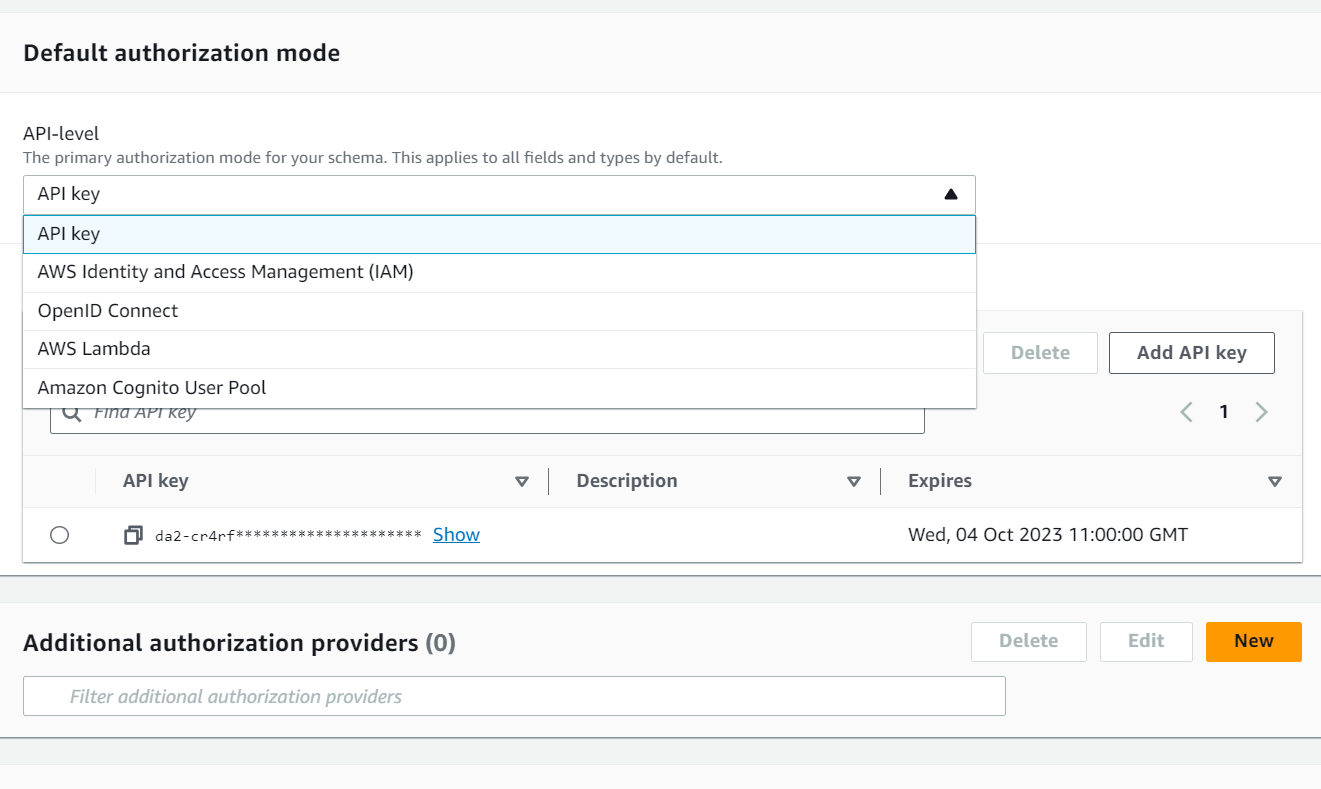
AWS_LAMBDA:For using an AWS Lambda function.
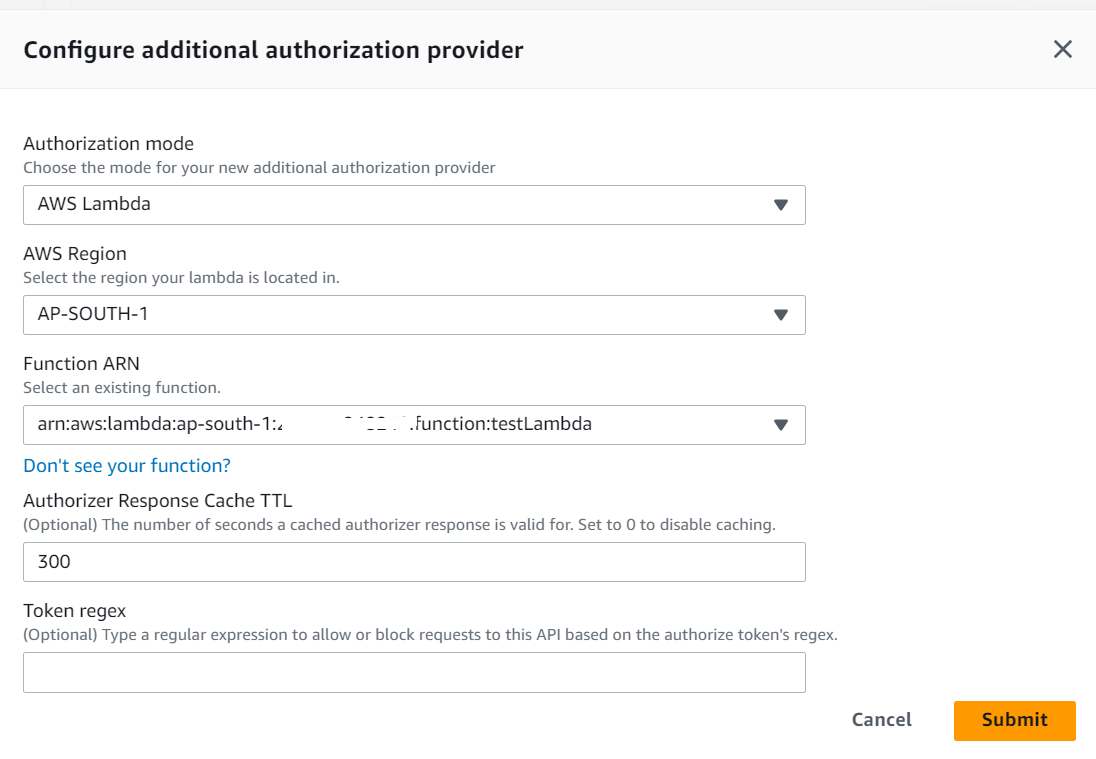
AWS_IAM:For using AWS Identity and Access Management (IAM) permissions.
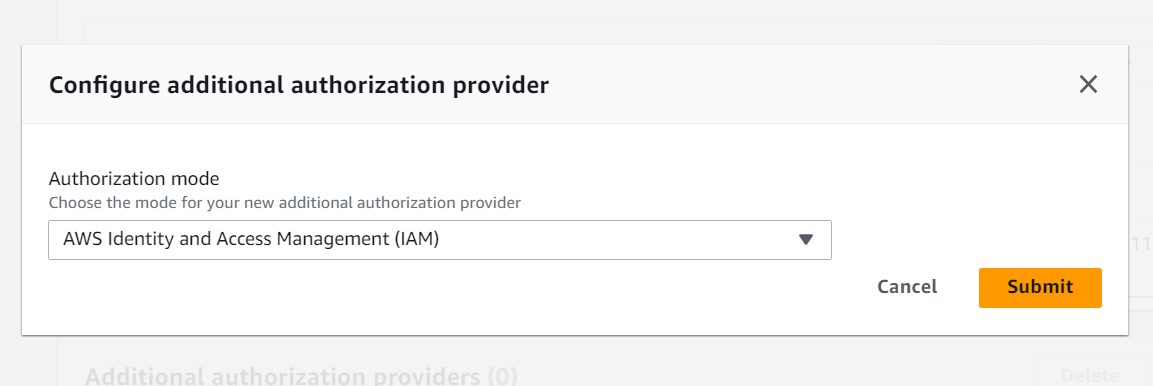
OPENID_CONNECT: For using your OpenID Connect provider.
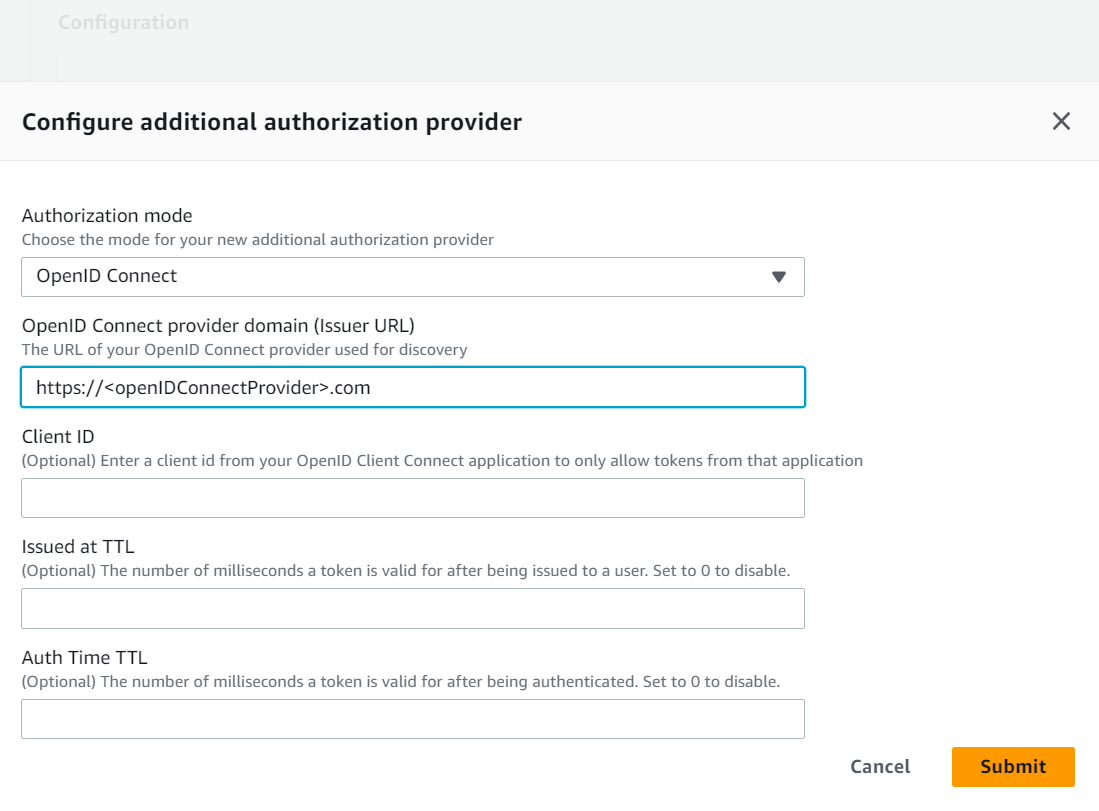
AMAZON_COGNITO_USER_POOLS: For using an Amazon Cognito user pool.
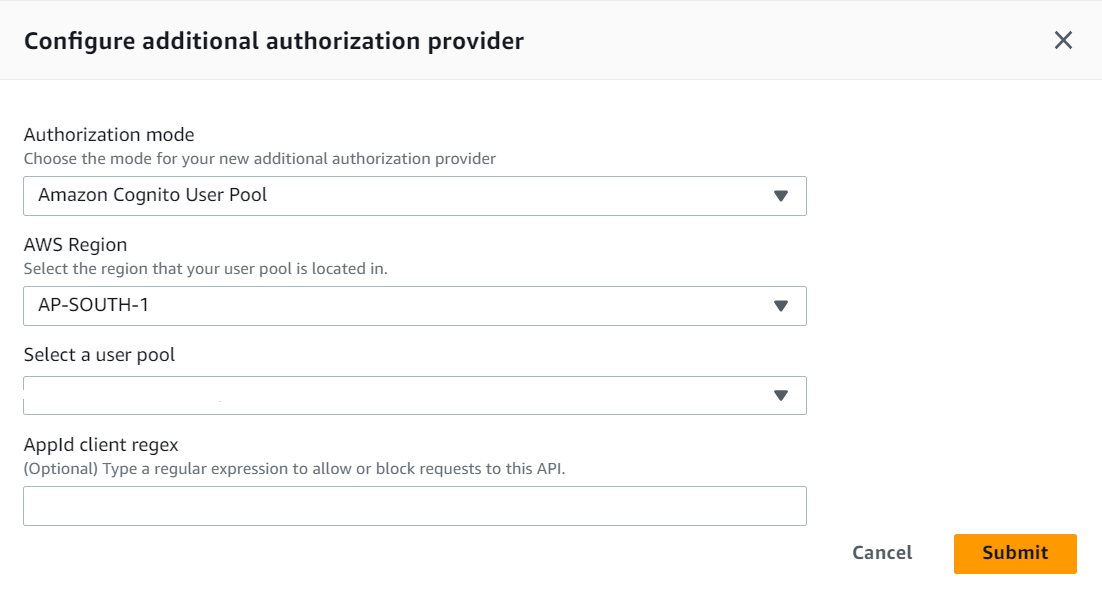
These basic authorization types work for most developers. For more advanced use cases, you can add additional authorization modes through the console, the CLI, and AWS CloudFormation. For additional authorization modes, AWS AppSync provides an authorization type that takes the values listed above (that is, API_KEY, AWS_LAMBDA, AWS_IAM, OPENID_CONNECT, and AMAZON_COGNITO_USER_POOLS).
When you specify API_KEY,AWS_LAMBDA, or AWS_IAM as the main or default authorization type, you can’t specify them again as one of the additional authorization modes. Similarly, you can’t duplicate API_KEY, AWS_LAMBDA or AWS_IAM inside the additional authorization modes. You can use multiple Amazon Cognito User Pools and OpenID Connect providers. However, you can’t use duplicate Amazon Cognito User Pools or OpenID Connect providers between the default authorization mode and any of the additional authorization modes. You can specify different clients for your Amazon Cognito User Pool or OpenID Connect provider using the corresponding configuration regular expression.
API Logging
Steps to Enable API Logging for an AppSync API:
- **Access the AWS AppSync Console**:
– Sign in to the AWS Management Console and open the AWS AppSync console.
- **Select Your API**:
– From the APIs page in the console, click on the name of the GraphQL API you want to enable logging for.
- **Access API Settings**:
– On your API’s homepage, go to the navigation pane and select “Settings.”
By default logging is disabled .
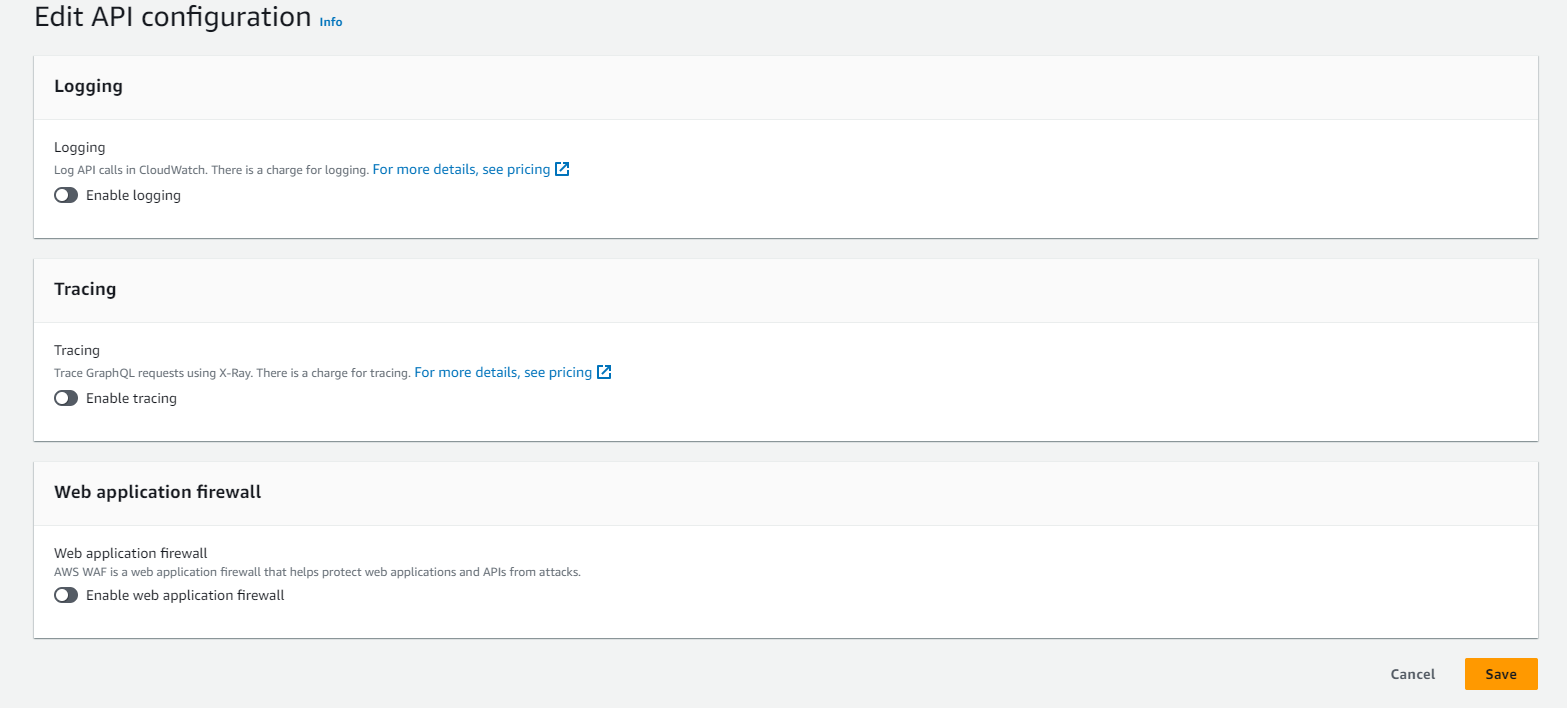
- **Enable Logging**:
– Under the “Logging” section, turn on “Enable Logs.”
- **Verbose Logging (Optional)**:
– If you want more detailed request-level logging, you can select the checkbox under “Include verbose content” (this step is optional).
- **Field Resolver Log Level (Optional)**:
– You can choose your preferred level of field-level logging (None, Error, or All) under “Field resolver log level” (optional).
- **Choose Role**:
– You have two options for specifying the role that allows AWS AppSync to write logs to CloudWatch:
– Create a new AWS Identity and Access Management (IAM) role by selecting “New role.”
– Use an existing IAM role by choosing “Existing role” and selecting the role’s Amazon Resource Name (ARN).
- **Save**:
– Click the “Save” button to apply the changes.
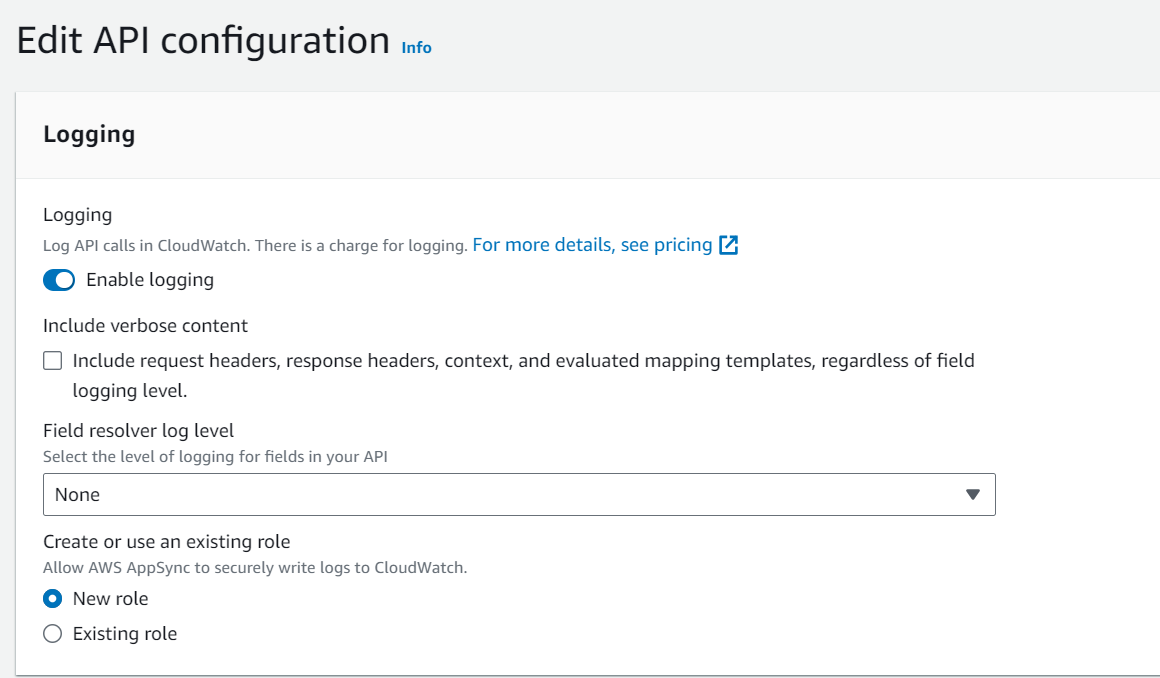
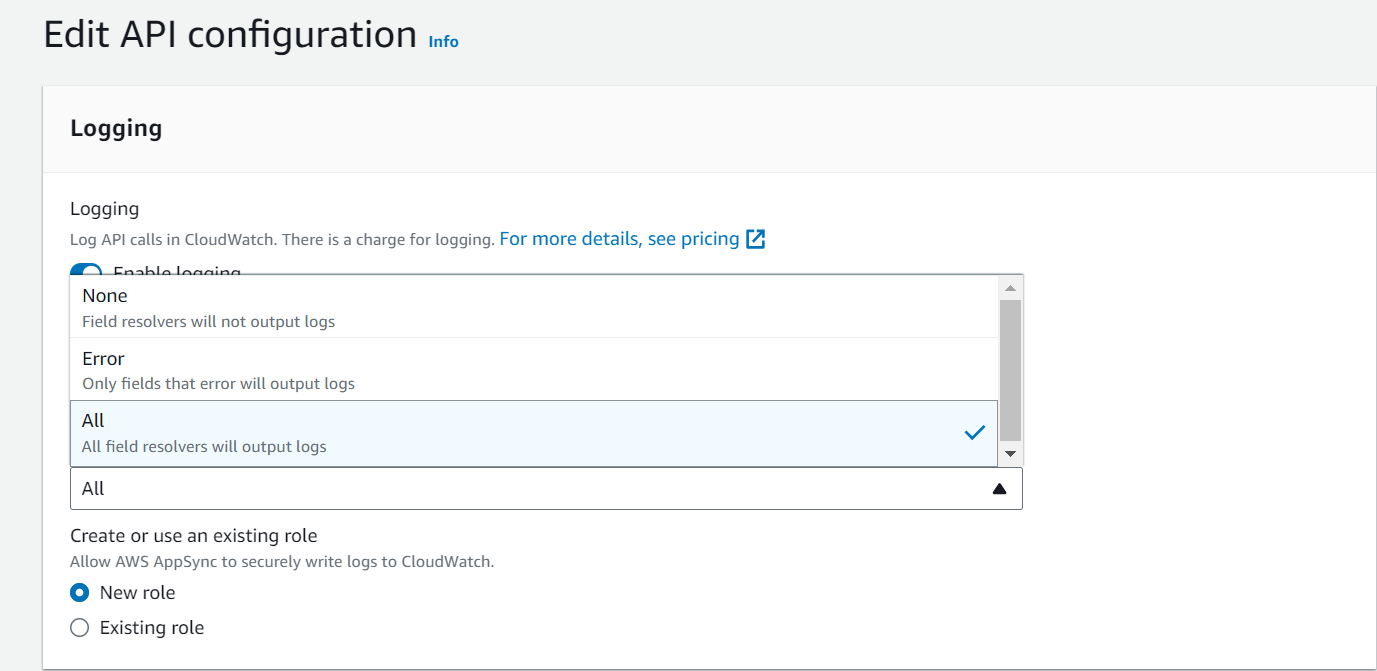
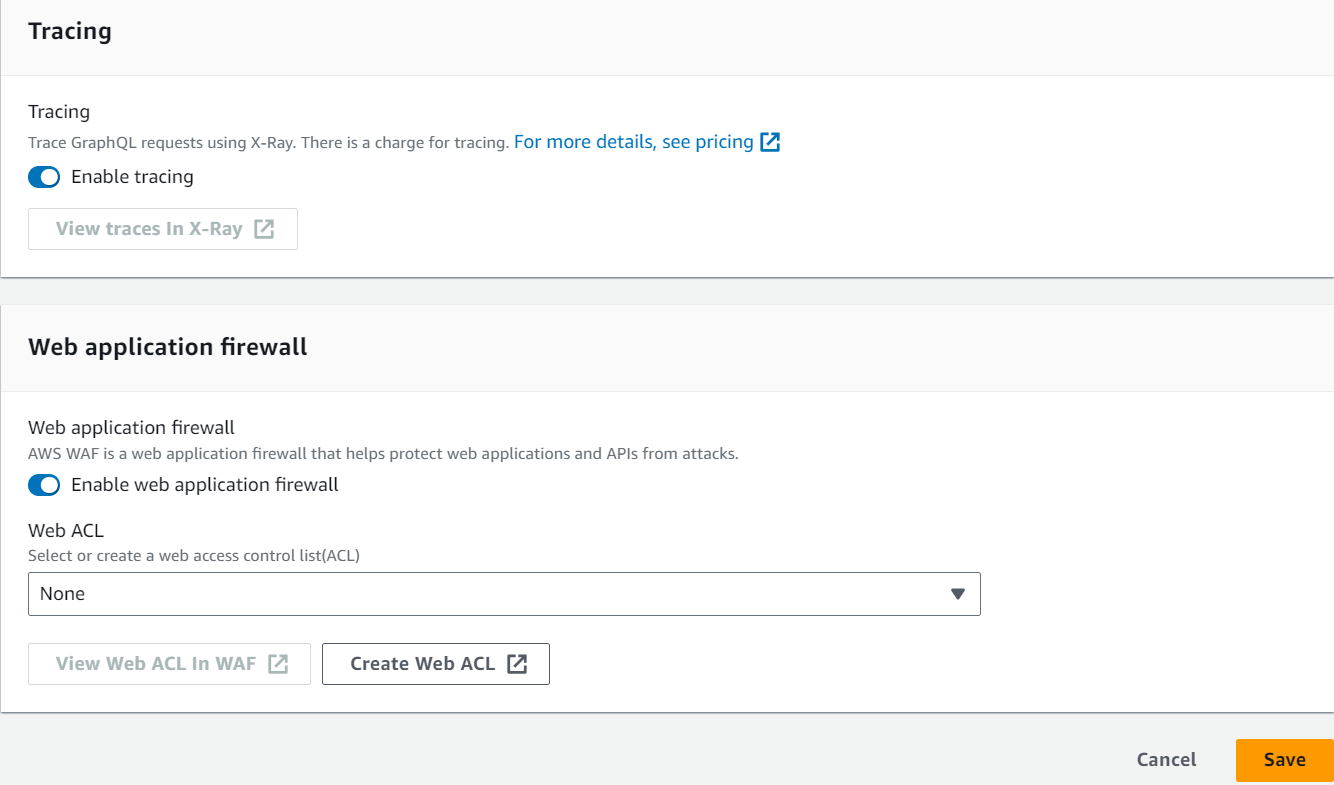
If you choose to use an existing IAM role, ensure that it has the necessary permissions to write logs to CloudWatch. AWS AppSync should be able to assume this role when logging data.
AWS AppSync logging is an important aspect of monitoring and maintaining your GraphQL APIs. It allows you to capture and analyze data about API requests, responses, errors, and custom application-specific events. Integration with Amazon CloudWatch provides a powerful platform for log analysis and real-time monitoring, helping you ensure the reliability and performance of your AppSync APIs.
API Caching and Compression:
AWS AppSync Data Caching:
– AWS AppSync has a built-in data caching system that stores data in a high-speed, in-memory cache.
– This cache makes data retrieval much faster, reducing the need to repeatedly access data sources like databases.
– Caching is available for both unit and pipeline resolvers, helping improve performance and reduce latency.
Compression for Faster Data Transfer:
– AWS AppSync also provides the option to compress API responses, making data load and download faster.
– This not only speeds up data transfer but can also reduce the load on your applications and potentially save on data transfer costs.
– You can configure compression settings as needed.
In summary, AWS AppSync’s data caching and compression features are designed to enhance the speed and efficiency of your API, making it faster and more cost-effective.
Types of caching:
1. None:
- No server-side caching is used.
- Every request goes directly to the data source without caching.
- No data is stored for reuse.
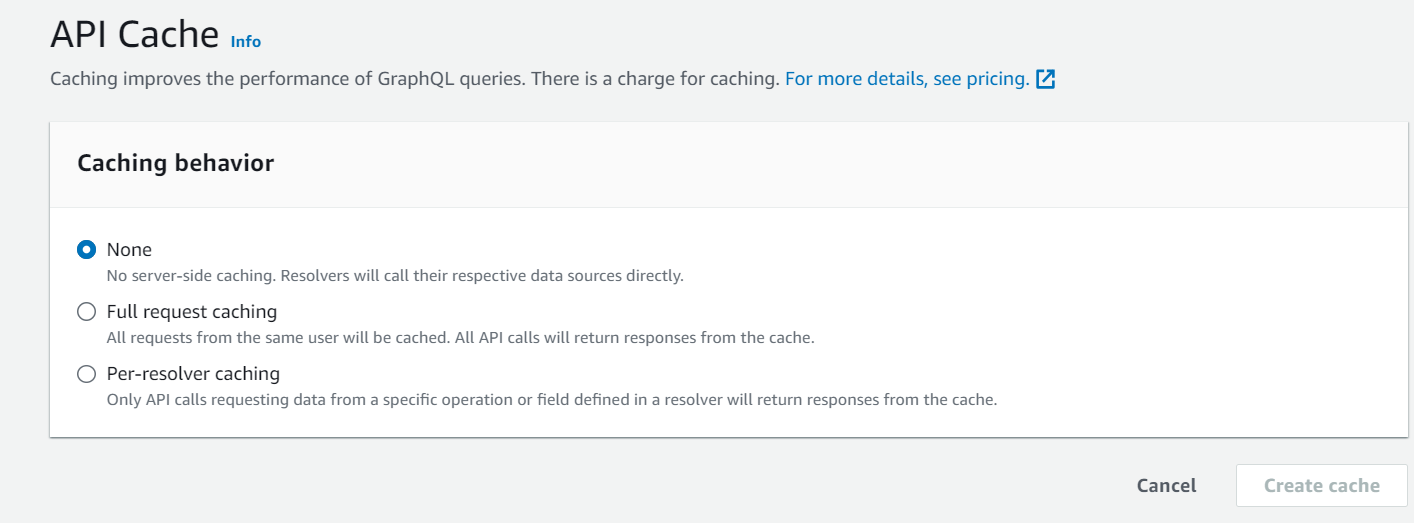
2. Full Request Caching:
- When data is fetched, it’s stored in a cache until it expires (based on Time-to-Live or TTL).
- Subsequent requests for the same data are served from the cache without contacting the data source again.
- Caching keys are typically based on request details like arguments and identity.
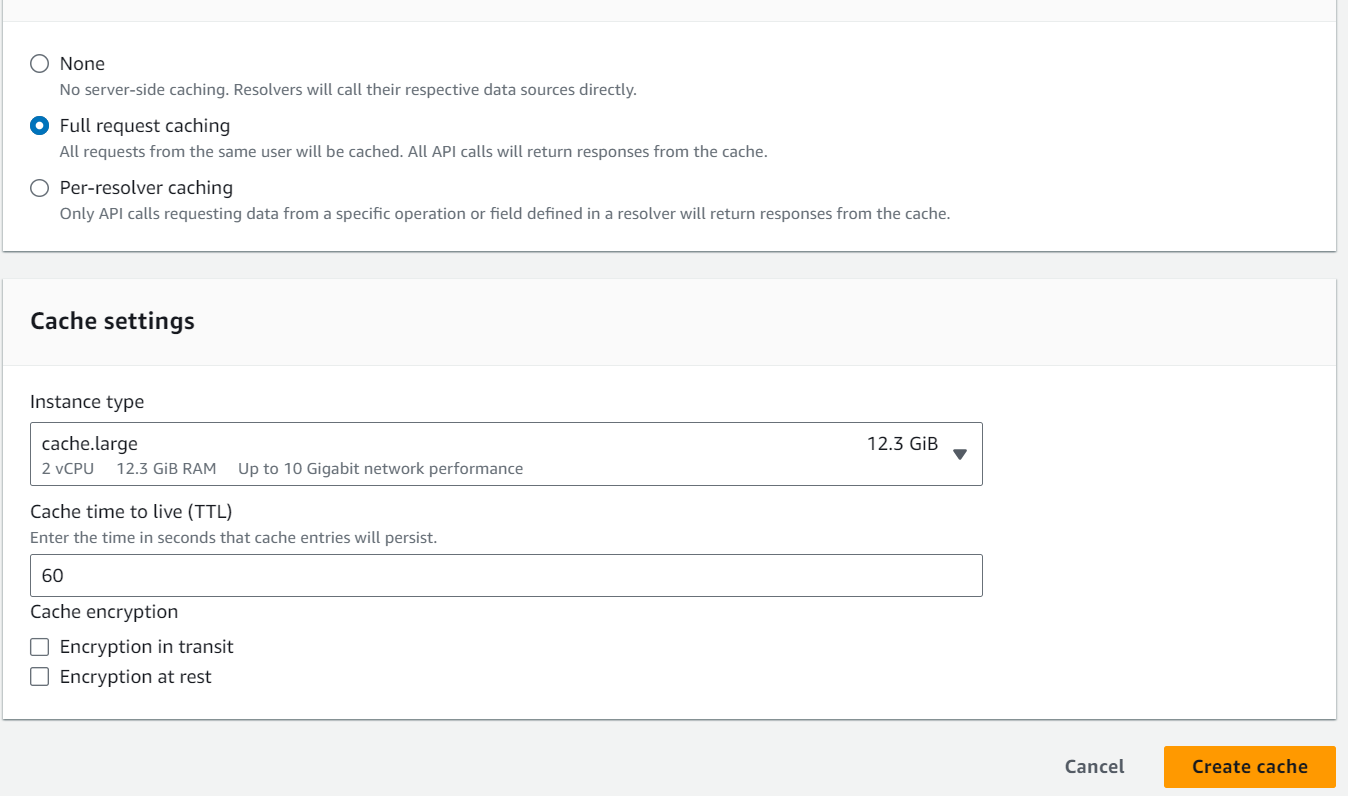
3. Per-Resolver Caching:
- Caching is specific to each resolver (part of a GraphQL query).
- Each resolver can choose to cache its responses or not.
- You can set a TTL (mandatory) and optional caching keys for each resolver.
- Caching keys can include details like request arguments, source data, or identity.
- If no caching keys are specified, default keys based on request context are used.
- In summary, AWS AppSync offers different caching behaviors. “None” means no caching, “Full Request Caching” caches entire requests, and “Per-Resolver Caching” allows resolvers to decide whether and how to cache their responses with customizable TTL and keys. These options help optimize API performance and data retrieval based on your specific requirements.
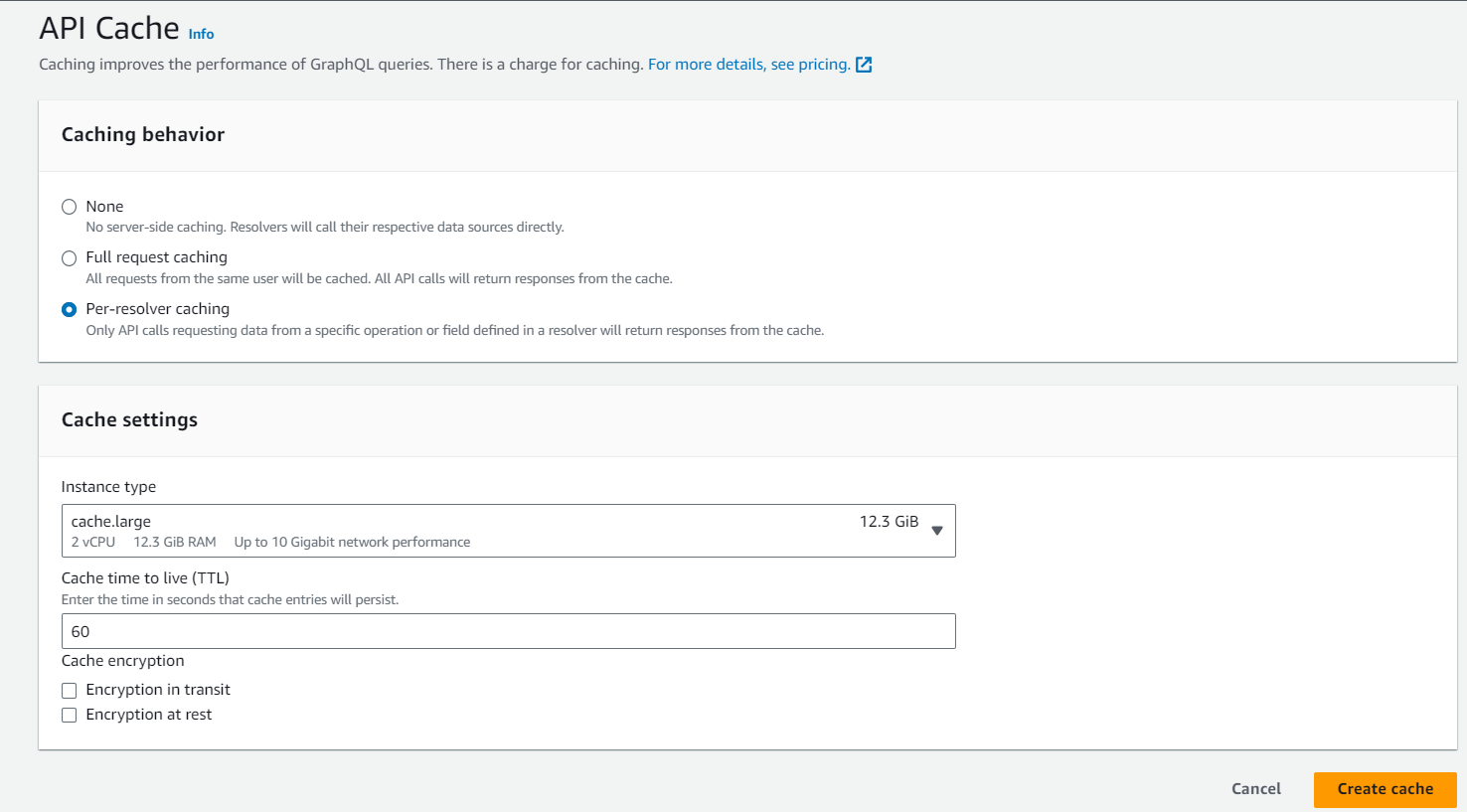
Merged API
As the use of GraphQL expands within an organization, trade-offs between API ease-of-use and API development velocity can arise. One the one hand, organizations adopt AWS AppSync and GraphQL to simplify application development by giving developers a flexible API they can use to securely access, manipulate, and combine data from one or more data domains with a single network call. On the other hand, teams within an organization that are responsible for the different data domains combined into a single GraphQL API endpoint may want the ability to create, manage, and deploy API updates independent of each other in order to increase their development velocities.
To resolve this tension, the AWS AppSync Merged APIs feature allows teams from different data domains to independently create and deploy AWS AppSync APIs (e.g., GraphQL schemas, resolvers, data sources, and functions), that can then be combined into a single, merged API. This gives organizations the ability to maintain a simple to use, cross domain API, and a way for the different teams that contribute to that API the ability to quickly and independently make API updates.
Steps to Create Merged API:
Log in to the AWS Management Console:
Make sure you have an AWS account and access to the AWS Management Console. Log in using your credentials.
Open AWS AppSync:
Navigate to the AWS AppSync service in the AWS Console by searching for “AppSync” in the AWS services search bar.
Step 1: In the Dashboard , choose Create API.Choose Merged API, then choose Next.
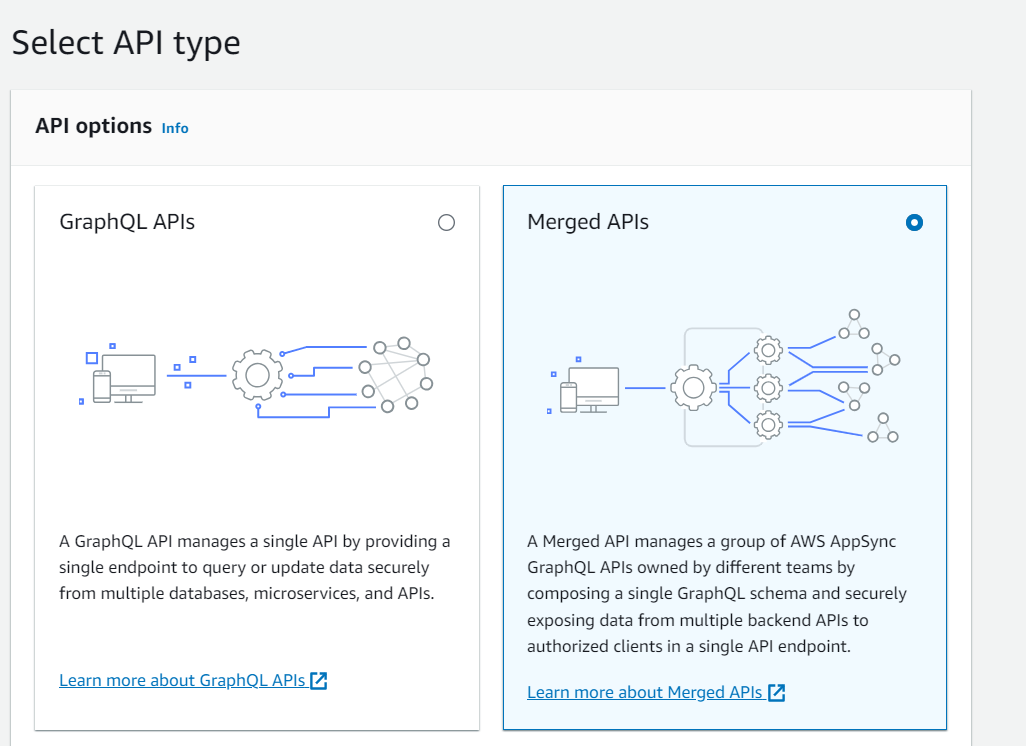
Step2:In the Specify API details page, enter in the following information:
Under API Details, enter in the following information:
Specify your merged API’s API name. This field is a way to label your GraphQL API to conveniently distinguish it from other GraphQL APIs.
Specify the Contact details. This field is optional and attaches a name or group to the GraphQL API. It’s not linked to or generated by other resources and works much like the API name field.
Under Service role, you must attach an IAM execution role to your merged API so that AWS AppSync can securely import and use your resources at runtime. You can choose to Create and use a new service role, which will allow you to specify the policies and resources that AWS AppSync will use. You can also import an existing IAM role by choosing Use an existing service role, then selecting the role from the drop-down list.
Under Private API configuration, you can choose to enable private API features. Note that this choice cannot be changed after creating the merged API. For more information about private APIs, see Using AWS AppSync Private APIs.
Choose Next after you’re done.
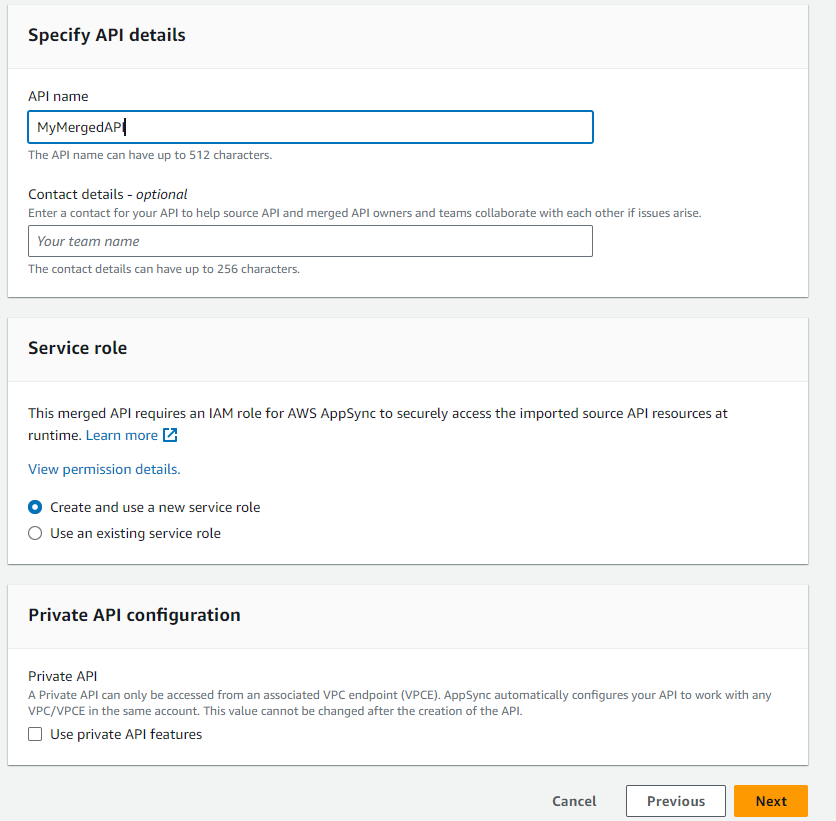
Step 3 :Next, you must add the GraphQL APIs that will be used as the foundation for your merged API. In the Select source APIs page, enter in the following information:
In the APIs from your AWS account table, choose Add Source APIs. In the list of GraphQL APIs, each entry will contain the following data:
Name: The GraphQL API’s API name field.
API ID: The GraphQL API’s unique ID value.
Primary auth mode: The default authorization mode for the GraphQL API. For more information about authorization modes in AWS AppSync.
Additonal auth mode: The secondary authorization modes that were configured in the GraphQL API.
Choose the APIs that you will use in the merged API by selecting the checkbox next to the API’s Name field. Afterwards, choose Add Source APIs. The selected GraphQL APIs will appear in the APIs from your AWS accounts table.
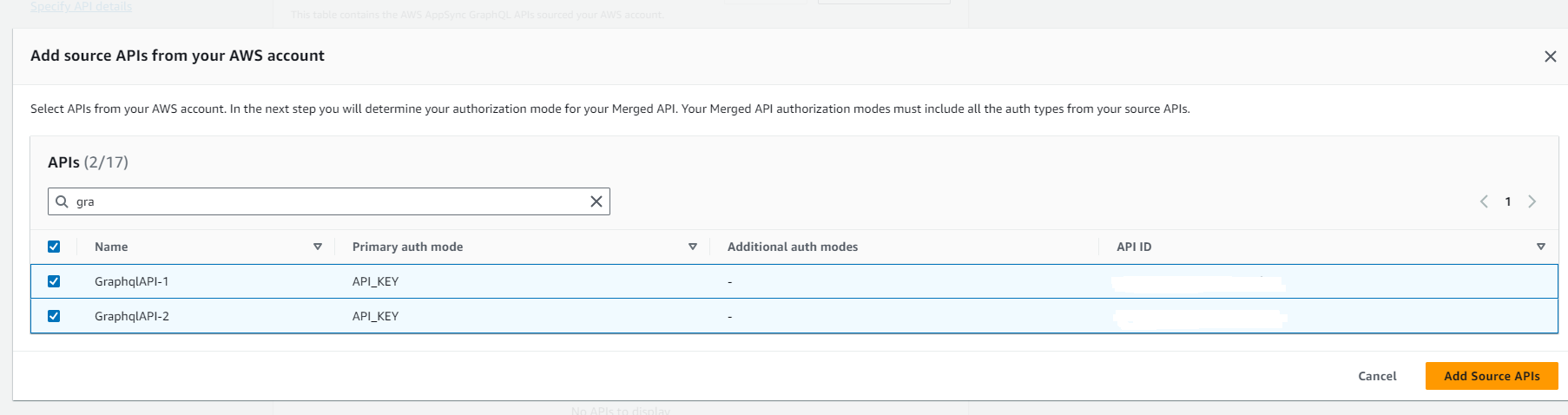
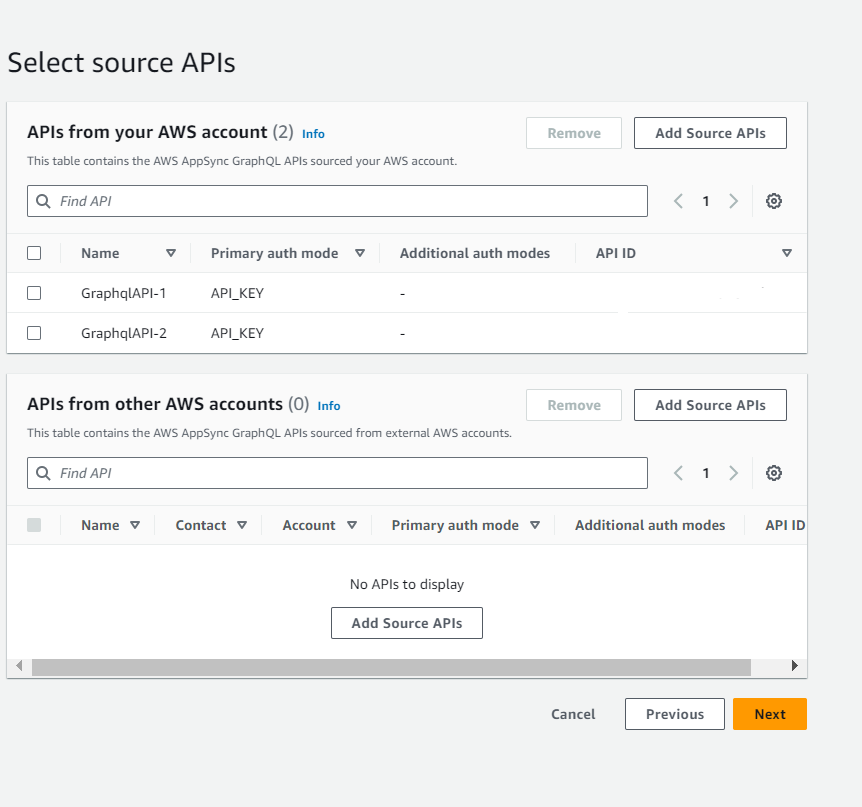
In the APIs from other AWS accounts table, choose Add Source APIs. The GraphQL APIs in this list come from other accounts that are sharing their resources to yours through AWS Resource Access Manager (AWS RAM). The process for selecting GraphQL APIs in this table is the same as the process in the previous section. For more information about sharing resources through AWS RAM, see What is AWS Resource Access Manager?.
Choose Next after you’re done.
Step 4 :Add your primary auth mode. See Authorization and authentication for more information. Choose Next.
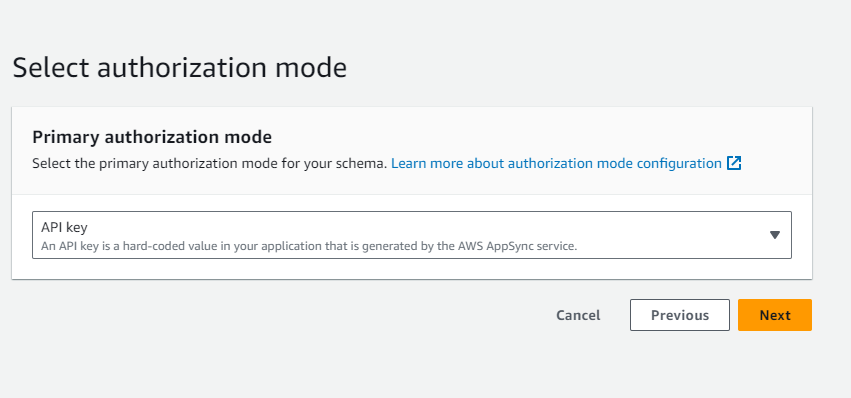
Step 5 :Review your inputs, then choose Create API.
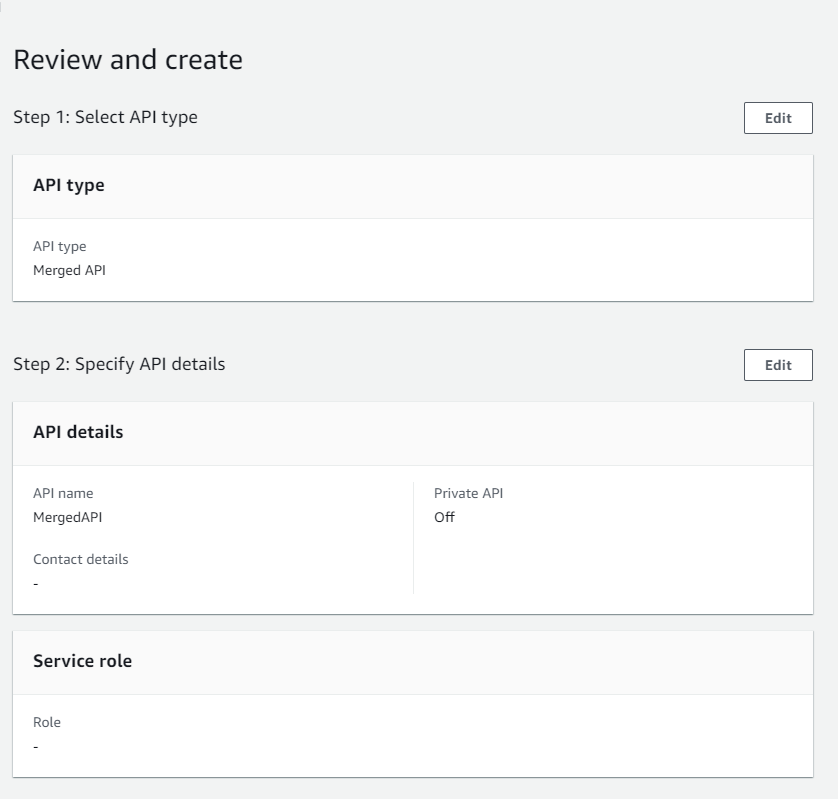
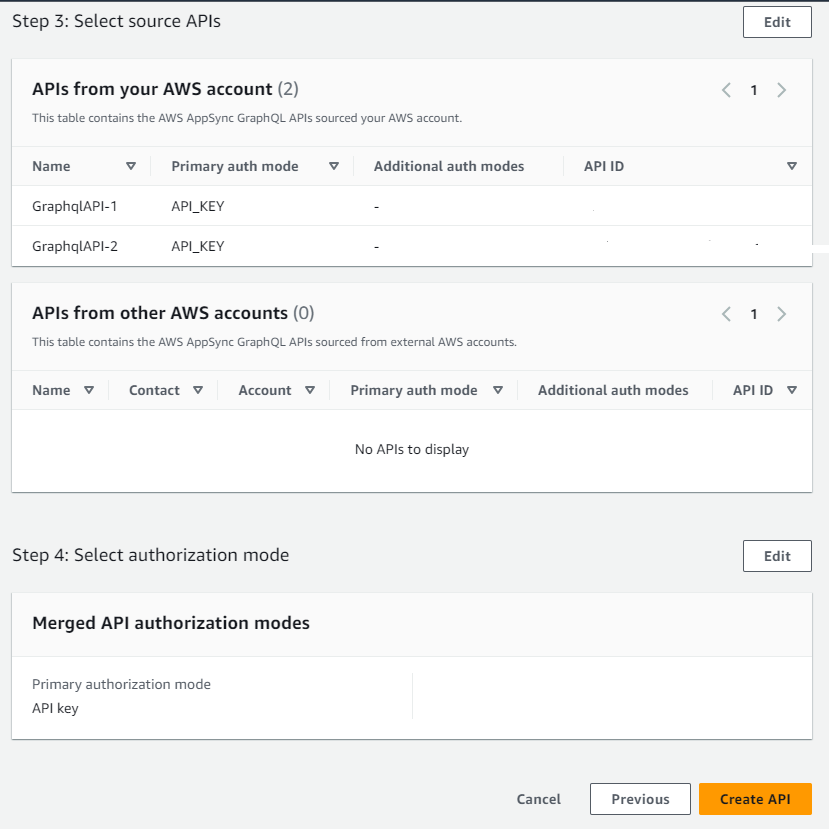
Step 6 : After creating merged API..it automatically merges the source API
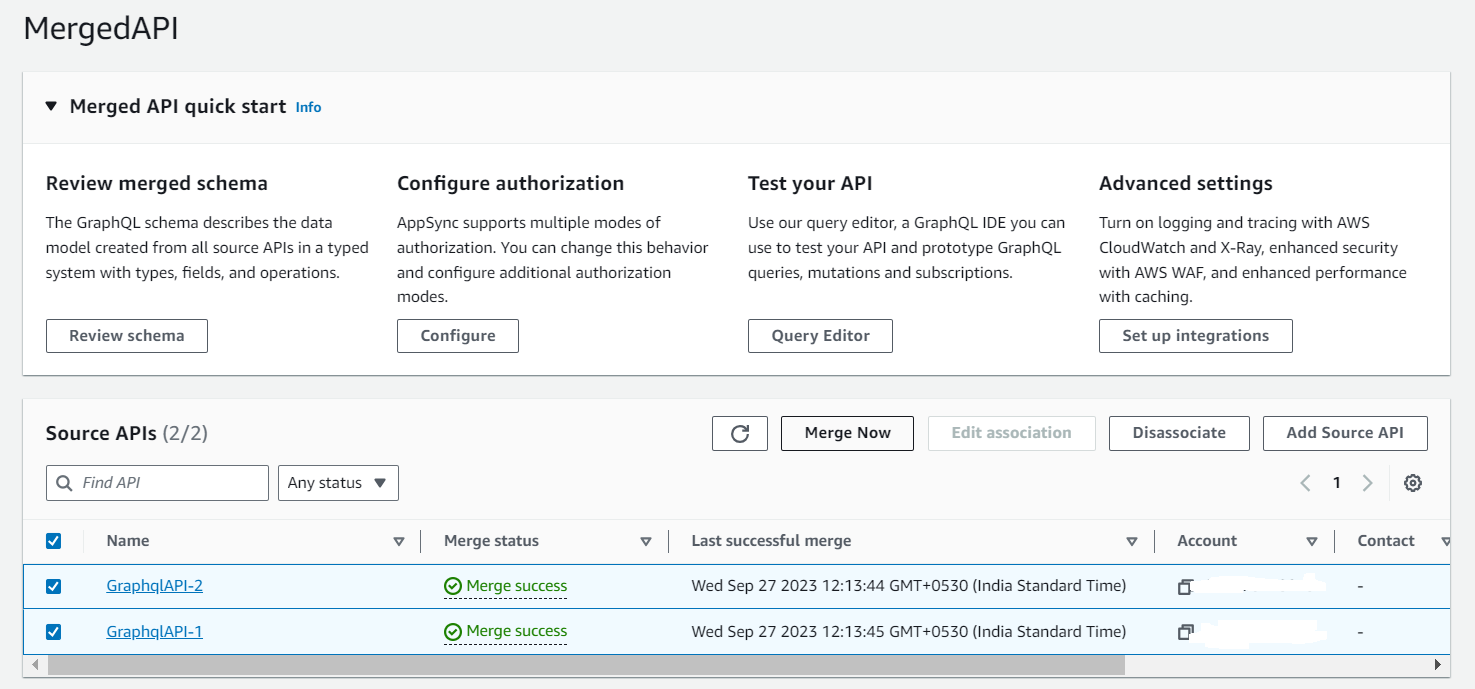
Merged API schema directives
AWS AppSync has introduced several GraphQL directives that can be used to- reduce or resolve conflicts across source APIs:
@canonical: This directive sets the precedence of types/fields with similar names and data. If two or more source APIs have the same GraphQL type or field, one of the APIs can annotate their type or field as canonical, which will be prioritized during the merge. Conflicting types/fields that aren’t annotated with this directive in other source APIs are ignored when merged.
@hidden: This directive encapsulates certain types/fields to remove it from the merging process. Teams may want to remove or hide specific types or operations in the source API so only internal clients can access specific typed data. With this directive attached, types or fields are not merged into the Merged API.
@renamed: This directive changes the names of types/fields to reduce naming conflicts. There are situations where different APIs have the same type or field name. However, they all need to be available in the merged schema. A simple way to include them all in the Merged API is to rename the field to something similar but different.
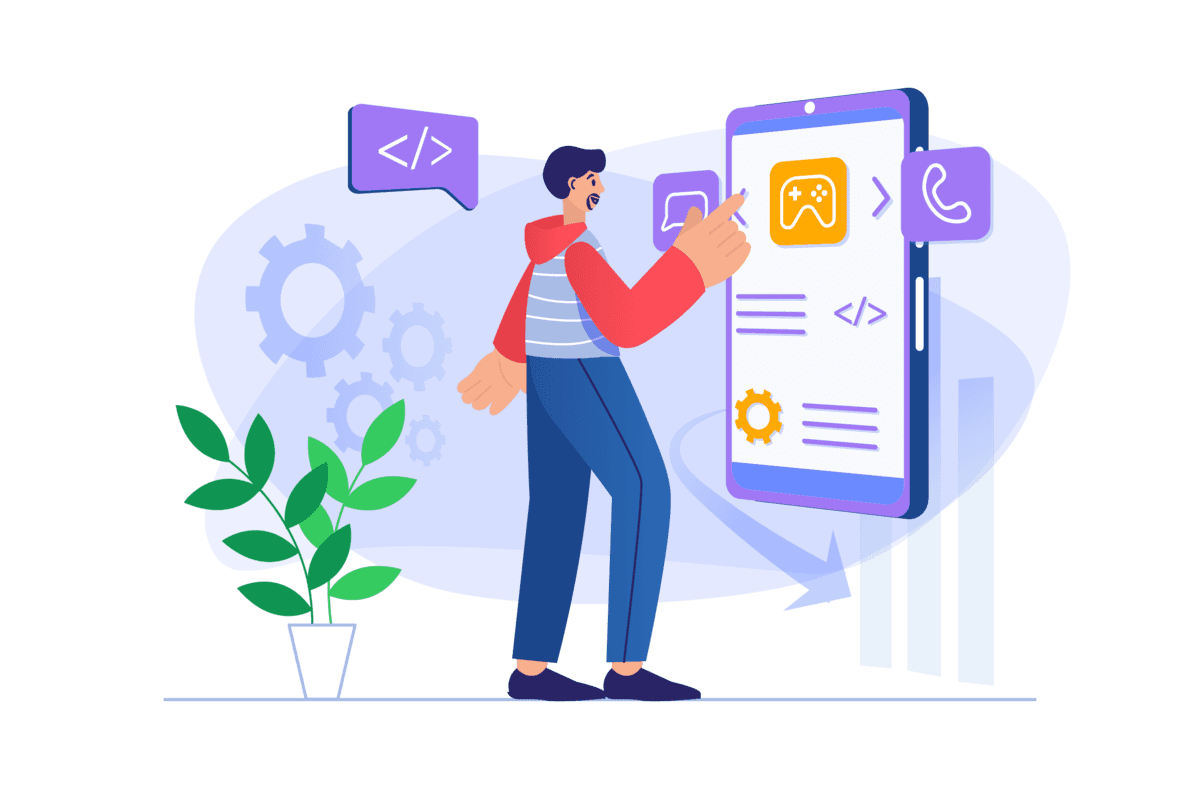
Building a Multilingual short News mobile app and admin panel with AWS Cloud Backend for Android and iOS Platforms
The client, a news media organization, wanted to develop a mobile application for Android and iOS platforms that would provide short news from India, AP & Telangana in multiple languages, such as English and Telugu. The application would also have a job search feature for both central/state/private jobs with search and filter and facets functionality, and a video section with entertainment, viral, movie, or interesting videos from across the web, integrated with YouTube and own videos.